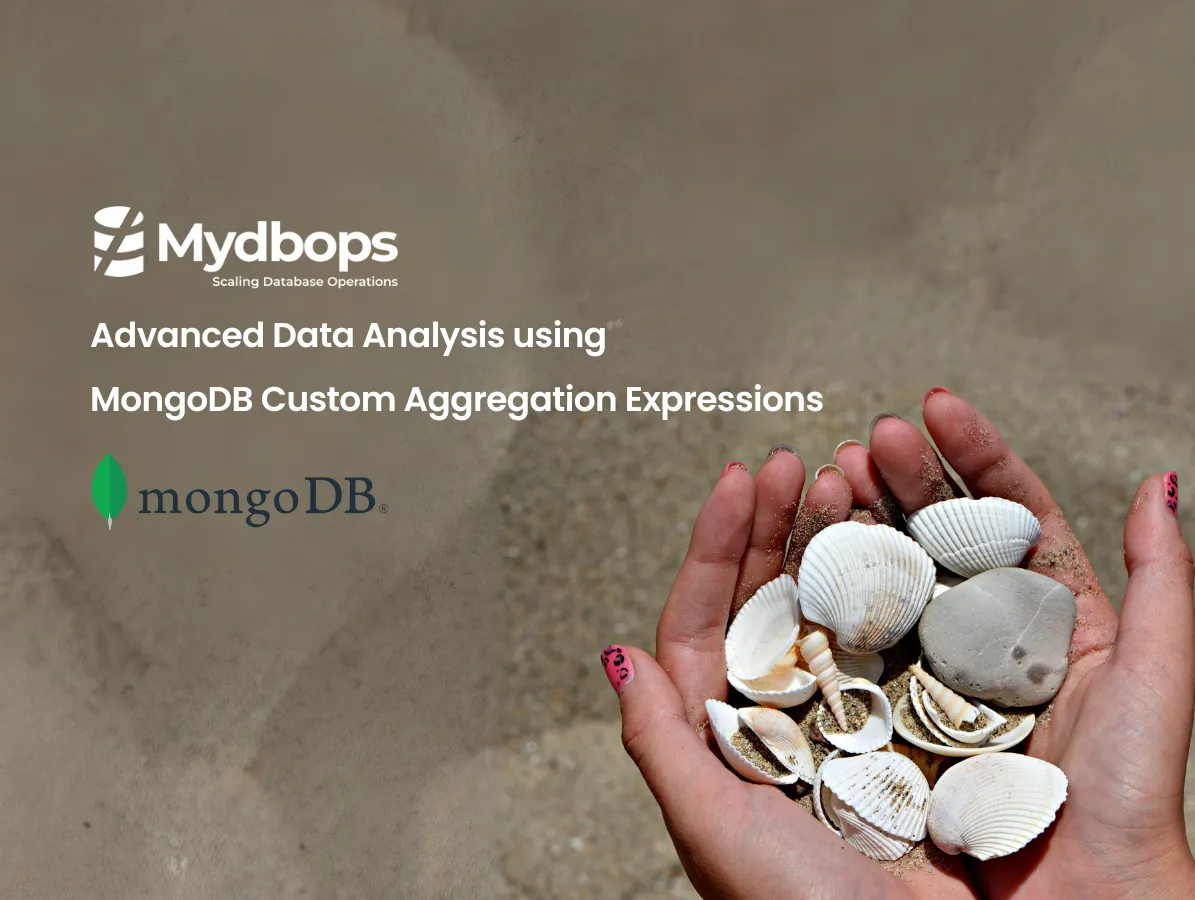
MongoDB, a leading NoSQL database, just got even more powerful with the introduction of custom aggregation expressions. By harnessing the $function and $accumulator operators, you can write your own JavaScript functions to perform complex data manipulations directly within your database. These tools can enhance performance, streamline workflows, and empower developers to tackle unique data challenges with ease. Let's unlock the full potential of MongoDB’s custom aggregation capabilities.
Custom Aggregation Expressions in MongoDB 4.4
Custom aggregation expressions in MongoDB 4.4 allow you to write JavaScript functions that seamlessly integrate into your aggregation pipelines. Whether you need to perform complex transformations or implement specific logic, these expressions give you the flexibility to tailor MongoDB to your needs.
Why Use Custom Aggregation Expressions?
- Using custom aggregation expressions can lead to better performance and increased developer productivity.
- By processing data directly within MongoDB, you can streamline your workflow and deliver faster results to your users.
- Plus, with the familiarity of JavaScript, you'll feel right at home crafting custom functions to tackle unique challenges.
Getting Started
Before we jump into coding, make sure you have MongoDB 4.4 installed. Familiarize yourself with the MongoDB Aggregation Framework if you haven't already, as it forms the foundation for custom expressions.
Understanding $function and $accumulator Operators
These operators are your go-to when you need to implement custom aggregation functions or expressions in MongoDB. Let's break down what they do:
$function: This operator lets you define custom JavaScript functions to implement behaviors not supported by MongoDB's Query Language. It's a handy tool when you need to tackle complex tasks that standard MongoDB functions can't handle.
$accumulator: This operator allows you to define custom accumulator functions with JavaScript. These functions maintain state as documents progress through the aggregation pipeline, offering flexibility for advanced data manipulation.
Considerations
Javascript Enablement
MongoDB provides server-side scripting capabilities using JavaScript, allowing you to run custom functions within aggregation queries. By default, this feature is enabled. However, if you're not using $function, $accumulator, $where, or MapReduce operators, you can enhance security and potentially improve performance by disabling server-side scripting.
Note:
For detailed information see security.javascriptEnabled configuration option or --noscripting command-line option.
Unsupported Array and String Functions
MongoDB 6.0 upgrades its internal JavaScript engine, which is responsible for executing server-side JavaScript code including $function, $accumulator, $where expressions and mapReduce operations. In this upgrade, several deprecated and non-standard array and string functions that existed in earlier versions are removed. It's essential to review the compatibility notes for MongoDB 6.0 to ensure that any custom JavaScript code you've written or dependencies you rely on are compatible with the new JavaScript engine.
Starting in MongoDB 6.0, the following array and string functions are removed and cannot be used in server-side JavaScript with $accumulator, $function, and $where expressions.
$function Operator
The $function operator empowers you to create custom JavaScript functions within your aggregation pipelines. Simply define your function body, specify any arguments, and let MongoDB handle the rest. From simple transformations to advanced computations, the possibilities are endless.
Syntax
Note:
Schema Validation Restriction: MongoDB allows you to define a schema for your collections to enforce data consistency and integrity. However, the $function operator cannot be used as part of schema validation query expressions. This means that you cannot use custom JavaScript functions to validate your schema.
Examples of “$function” Usage
Here is an example showcasing $function in a MongoDB aggregation pipeline, focusing on real-time product discount calculations:
Insert test data
Let's say we have two collections named "products" and “discounts” with documents that represent individual products, each containing their name, price and category:
Scenario: Calculate the discounted price for each product in an e-commerce store based on a dynamic discount percentage stored in a separate collection.
Aggregation pipeline
This example utilises $function to access the discount information from another collection and apply it to the product price in the current collection.
Explanation
- In this example, the $function operator is used within the $project stage to calculate the discounted price for each product. It takes two arguments:
- price: The original price of the product from the products collection.
- discount: The discount percentage obtained from the discountInfo array after the $lookup and $unwind stages (may be null if no matching discount exists).
- The function checks if a discount is available. If not, it returns the original price. Otherwise, it calculates the discounted price by multiplying the original price by (1 - discount / 100), effectively applying the discount as a percentage.
This is just one example of how $function can be used for complex calculations within MongoDB aggregations. You can adapt this concept to various scenarios where you need to perform dynamic data manipulation based on custom logic.
After running this aggregation pipeline, the resulting documents will have an additional field called "discount", containing the average score calculated for each product.
$accumulator Operator
For more complex scenarios requiring stateful processing, the $accumulator operator comes to the rescue. This operator allows you to define custom accumulator functions that maintain state as documents flow through the pipeline. Whether you're aggregating data over time or performing intricate calculations, the $accumulator operator has you covered.
Syntax
Note:
Usage Restriction: $accumulator is available in these stages:
Examples of “$accumulator” Usage
Insert test data
Let's say we have a collection named "students" with documents that represent individual students, each containing their user name and an array of their exam marks:
Scenario: Calculate the statistical data of a Stoke website for analysis of the Stokes.
Aggregation pipeline
Explanation
- $project: This stage projects only the "WeeklyRate" and "name" fields from each document, discarding other fields.
- $unwind: This stage deconstructs the "WeeklyRate" array field, creating a new document for each element in the array. This allows for easier aggregation and analysis of individual weekly rates.
- $group: This stage groups the documents by the "name" field, creating groups of documents with the same name. Within each group, it applies the $accumulator operator to calculate various statistics for the weekly rates.
- $accumulator: This custom accumulator operator is used to calculate statistics such as count, sum, sum of squares, minimum, maximum, mean, variance, and standard deviation for the weekly rates within each group.
- init: Initializes the accumulator with initial values for count, sum, sum of squares, minimum, and maximum.
- accumulate: Accumulates values from the "WeeklyRate" field into the accumulator, updating count, sum, sum of squares, minimum, and maximum.
- accumulateArgs: Specifies the field from which to extract values for accumulation, in this case, the "WeeklyRate" field.
- merge: Merges two accumulators together during the aggregation process, combining their counts, sums, sum of squares, minimums, and maximums.
- finalize: Finalizes the accumulator by calculating the mean, variance, and standard deviation based on the accumulated values.
- $accumulator: This custom accumulator operator is used to calculate statistics such as count, sum, sum of squares, minimum, maximum, mean, variance, and standard deviation for the weekly rates within each group.
This pipeline outputs statistics such as count, sum, sum of squares, minimum, maximum, mean, variance, and standard deviation for each group of weekly rates, grouped by the "name" field. These statistics provide insights into the distribution and variability of weekly rates within each group.
With MongoDB's custom aggregation expressions, you have the power to unlock new possibilities for data manipulation and analysis. Whether you're a seasoned MongoDB developer or just getting started, these features provide a powerful toolkit to elevate your projects to the next level.
Ready to unlock the full potential of MongoDB for your data manipulation and analysis needs? Partner with Mydbops for expert MongoDB Managed, Consulting, and Remote DBA Services. Our team of experienced DBAs can help you leverage MongoDB's advanced features and optimize your database performance. Contact Mydbops today.
{{cta}}