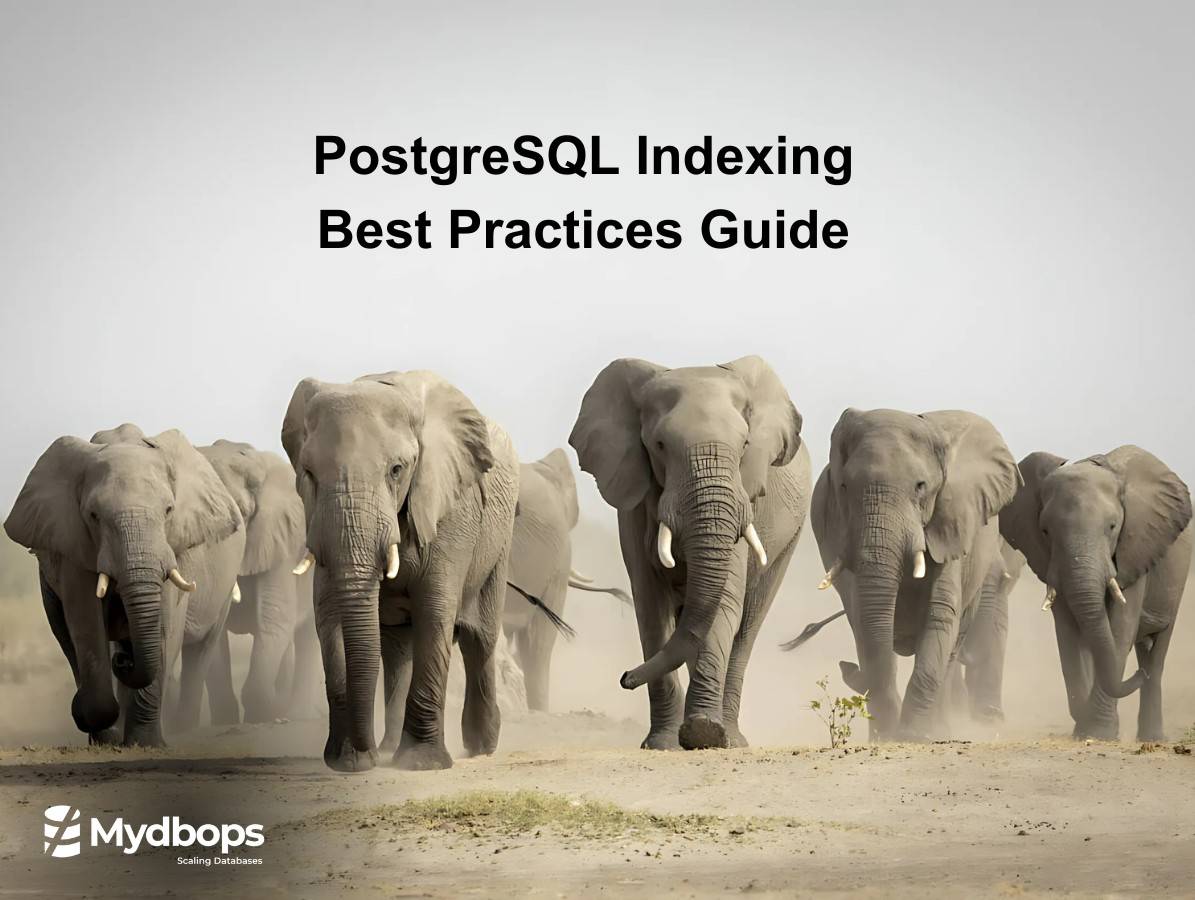
PostgreSQL Indexing Best Practices: Boosting Query Performance Effectively
Indexes are like the secret weapon of PostgreSQL—they can dramatically speed up your queries, making data retrieval lightning-fast. But here’s the catch: while a well-planned indexing strategy can make your database incredibly efficient, improper indexing can slow things down, waste storage, and even hurt performance.
So how do you strike the right balance?
This blog dives deep into PostgreSQL indexing best practices—covering index types, when to use them, common pitfalls, and expert tips to optimize them effectively. By the end, you’ll know exactly how to use indexes to supercharge your queries while keeping your database lean and efficient.
Types of Indexes in PostgreSQL
PostgreSQL offers several types of indexes, each designed for specific use cases. Understanding these types will help us choose the right index for our queries.
For more details, refer to the PostgreSQL documentation
B-Tree Index
Think of a library's catalog where books are sorted alphabetically. The B-Tree Index works similarly—it organizes data in a balanced tree structure, making searches efficient.
CREATE INDEX idx_employee_id ON employee_records (employee_id);
- Default and most commonly used index type.
- Optimizes ORDER BY and DISTINCT queries by maintaining sorted data for faster sorting and unique value retrieval.
- Efficient for equality (=) and range queries (<, >, BETWEEN, IN, IS NULL, IS NOT NULL).
- Works best with sorted data and columns with high cardinality (i.e., unique or widely varying values).
Hash Index
Imagine a hotel storing room keys in numbered lockers. If you know your locker number, retrieval is instant. Hash indexes store values in a way that makes exact matches extremely fast.
CREATE INDEX idx_employee_hash ON employee_records USING hash (employee_id);
- Supports only = (equality) comparisons.
- Faster than B-Tree for simple equality checks but does not support range queries (<, >, BETWEEN).
- Crash-safe and replicatable from PostgreSQL 10 onwards due to Write-Ahead Logging (WAL) support (PostgreSQL 10 Release Notes).
- It is best suited for high-speed lookups where only exact matches are needed.
BRIN Index (Block Range INdex)
For databases handling time-series data, like event logs, searching without an index can be slow. The BRIN Index works like a map—it stores only min-max value ranges for efficient scanning.
CREATE INDEX idx_brin_date ON logs USING brin (log_date);
- Best for very large tables with sequentially inserted data, such as time-series logs.
- Stores only min-max value ranges per block, reducing storage usage compared to B-Tree.
- Optimized for naturally ordered columns, like timestamps or auto-incremented IDs.
- Space-efficient and faster for range scans, but less precise than B-Tree for random lookups.
GIN Index (Generalized Inverted Index)
The Generalized Inverted Index (GIN) is designed for multi-value columns like arrays, JSONB, and full-text search.
CREATE INDEX idx_product_tags ON products USING GIN (tags);
- Best for multi-value columns like arrays, JSONB, and full-text search.
- Optimized for fast lookups in composite values using operators like @>, <@, ?, and &&.
- Efficient for containment and existence checks in structured data.
- Slower to create since it scans and processes the entire document upfront.
GiST Index (Generalized Search Tree)
If GIN is for keywords, GiST (Generalized Search Tree) is for geospatial and complex searches. Think of it as a navigator that efficiently indexes irregular data.
CREATE INDEX idx_location ON places USING gist (location);
- Efficient indexing for complex data types.
- Supports complex data types like geometric shapes, full-text search, and range queries.
- Uses a tree structure with flexible data matching.
- Ideal for spatial queries, nearest-neighbor searches, and similarity-based lookups.
SP-GiST Index (Space-Partitioned GiST)
SP-GiST works well for non-balanced data structures like quadtrees, k-d trees, and tries. Think of it as a roadmap for organizing unstructured, hierarchical, or sparse data.
CREATE INDEX idx_spatial ON locations USING spgist (coordinates);
- Supports non-balanced data structures like quadtrees, k-d trees, and tries for efficient indexing.
- Optimized for spatial searches and hierarchical data, such as geographic locations and network routing.
- Ideal for unstructured or sparse data, enabling fast lookups in datasets with irregular distributions.
- Provides efficient indexing for specialized use cases, including IP address searches and complex pattern matching.
When to Use Indexes in PostgreSQL
Indexes are powerful tools for improving query performance, but they should be used strategically. Here are key scenarios where indexing is beneficial:
- Frequent Filtering: Use indexes on columns frequently appearing in WHERE conditions to speed up lookups.
- Join Operations: Index foreign key columns to improve JOIN performance between large tables.
- Sorting & Grouping: Index columns used in ORDER BY and GROUP BY to avoid expensive sorting operations.
- Unique Constraints: Use unique indexes to enforce data integrity and accelerate searches.
- Full-Text Search: Use GIN or GiST indexes for efficient full-text search queries.
- Large Tables: Consider BRIN indexes for large tables with naturally ordered data to save storage.
- Partial Indexes: Use partial indexes to optimize queries that filter on specific conditions (e.g., active users).
- Expression Indexes: Index computed expressions to speed up queries that rely on function-based filtering.
- Specialized Data Types: Use GiST or SP-GiST for indexing geometric, network, or hierarchical data.
When to Avoid Excessive Indexing
- Frequent Updates/Inserts/Deletes: Indexing slows down writes as each change requires index maintenance.
- Small Tables: Sequential scans might be faster than using indexes.
- Low Cardinality Columns: Avoid indexing columns with very few unique values (e.g., boolean flags).
- Too Many Indexes: Maintaining multiple indexes on a table increases storage use and degrades write performance.
Best Practices for Creating and Managing Indexes
Choose the Right Index Type
- Select the index type based on the query patterns and data types.
Index Selective Columns
- Index columns with high cardinality (many unique values).
- Avoid indexing low-cardinality columns (e.g., boolean flags, gender).
- Use partial indexes to optimize queries that frequently filter on specific values.
CREATE INDEX idx_active_users ON users (status) WHERE status = 'active';
Use Composite Indexes for Multi-Column Queries
- Create multi-column indexes for queries filtering on multiple columns.
- Ensure the column order matches the query’s filter order.
CREATE INDEX idx_orders_customer_date ON orders (customer_id, order_date);
Use Expression Indexes for Complex Queries
- Expression indexes speed up queries on computed values or case-insensitive searches.
- Useful for UPPER, LOWER, date functions, JSONB expressions, etc.
CREATE INDEX idx_lower_email ON users (LOWER(email));
Avoid Over-Indexing
- Having too many indexes can slow down INSERT, UPDATE, and DELETE operations.
- Regularly monitor and remove unused indexes
Drop Duplicate Indexes to Reduce Overhead
- Duplicate indexes unnecessarily occupy storage and degrade performance.
- Drop unnecessary duplicate indexes.
Create Indexes Concurrently to Prevent Locking
- Creating an index locks the table, blocking writes.
- Use CONCURRENTLY to create indexes without downtime.
CREATE INDEX CONCURRENTLY idx_employee_department ON employees (department);
Rebuild Indexes to Prevent Bloat
- Over time, indexes grow in size and slow down. Use REINDEX to optimize them.
REINDEX INDEX idx_employee_salary;
Use Autovacuum to Maintain Index Health
- Ensure autovacuum is running to prevent index bloating.
Test and Benchmark Index Performance
- Always test queries using EXPLAIN ANALYZE before and after creating indexes.
EXPLAIN ANALYZE SELECT * FROM employees WHERE department = 'IT';
Common Pitfalls to Avoid with Indexes
- Over-Indexing: Too many indexes slow down inserts, updates, and overall performance.
- Wrong Index Type: Using a B-Tree for JSONB search instead of GIN reduces efficiency.
- Ignoring ANALYZE and VACUUM: Leads to outdated statistics and poor query plans.
- Not Using Composite Indexes: If filtering by multiple columns, create an index covering both.
- Unused Indexes: Regularly check and drop unused indexes to free up resources.
- Indexing Every Column: Creating indexes without a purpose increases storage and write overhead.
- Ignoring Index Maintenance: Failing to rebuild or vacuum indexes results in bloat and slow queries.
- Overlooking Query Patterns: Indexing without analyzing queries can lead to inefficiency.
- Neglecting Index Size: Large indexes consume memory and storage, affecting performance.
- Forgetting to Drop Unused Indexes: Unused indexes waste resources and slow down operations.
- Misordering Composite Index Columns: Ensure column order matches query filters for optimal performance.
- Ignoring Locking and Concurrency: Use CONCURRENTLY when creating indexes on large tables to prevent downtime.
Conclusion
Indexes are a powerful tool for optimizing query performance in PostgreSQL, but they require careful planning and management. Understanding different index types, applying them strategically, and following best practices such as avoiding over-indexing and regularly maintaining indexes can significantly enhance database efficiency. To ensure optimal performance, monitor index usage, test query execution plans, and make adjustments as needed. With a well-structured indexing strategy, PostgreSQL databases can handle large workloads efficiently and deliver high-performance queries.
Ensure efficiency, security, and scalability with Mydbops' expert services—from managed PostgreSQL solutions and query optimization to 24/7 support and security audits. Connect with us today.
{{cta}}