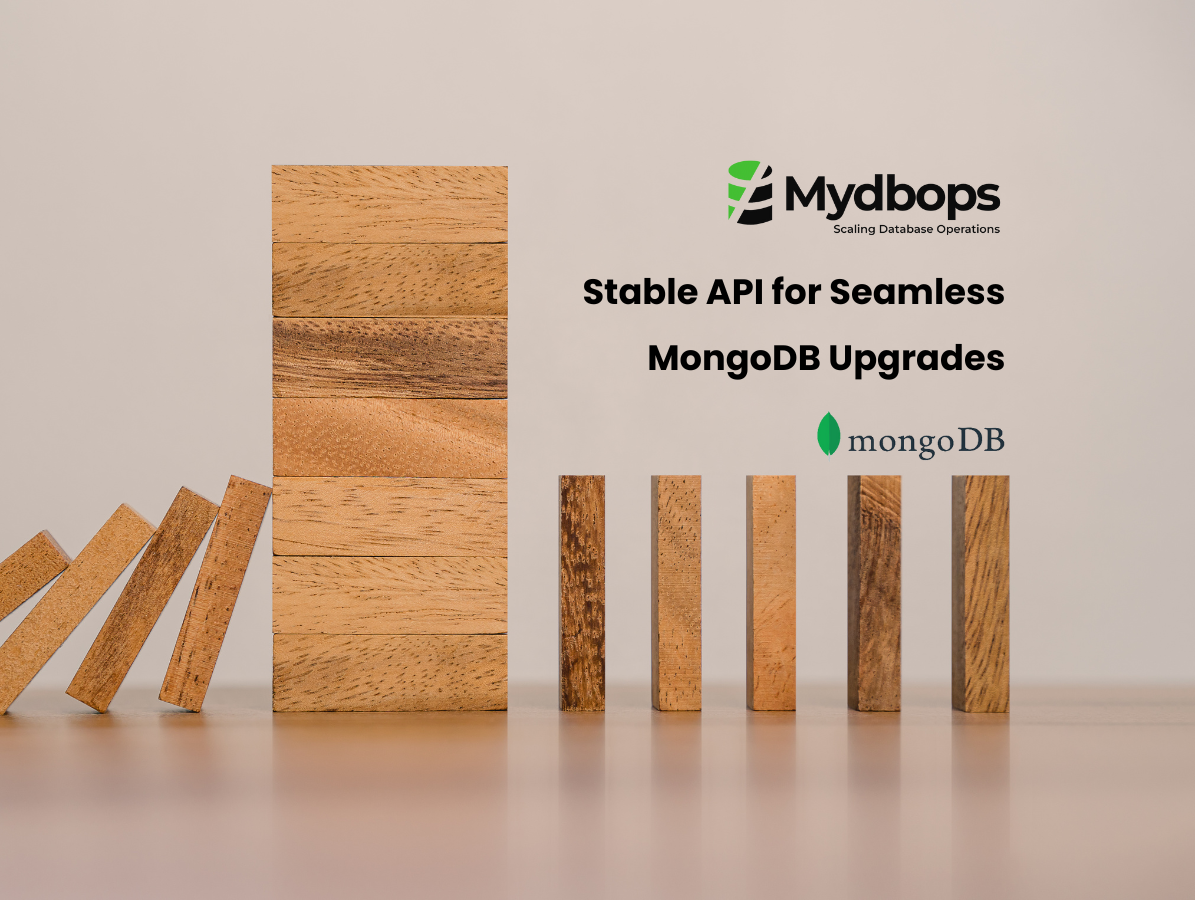
In one of our critical projects—a real-time logistics management platform—MongoDB served as the backbone for managing shipments, customer data, and order processing. As the system scaled and data loads increased, upgrading MongoDB to newer versions became essential. However, this posed a significant risk: potential downtime due to unexpected query failures or changes in MongoDB’s behavior.
The MongoDB Stable API provided the solution, allowing us to manage the upgrade process without the risk of breaking existing functionality. This was crucial to ensuring our platform continued to operate smoothly as we scaled and upgraded our infrastructure.
The Challenge: Preventing Downtime and Query Failures
In our client’s logistics platform, hundreds of transactions, queries, and data modifications took place every minute. Any unexpected change in query behavior during database upgrades could result in data inconsistencies, delayed shipments, and overall downtime, causing significant disruptions to operations.
The system could not afford:
- Query failures
- Changes in behavior
- Extended downtime
Solution: Leveraging MongoDB’s Stable API
MongoDB’s Stable API enabled us to lock the application’s behavior to a specific API version, ensuring that queries, updates, and other operations remained consistent even as we upgraded MongoDB.
Locking in the API Version
The first step in ensuring stability during MongoDB upgrades was locking the API version. MongoDB allows developers to specify an API version when issuing commands, ensuring that the database's behavior remains consistent across different releases.
In our case, we locked the system to Stable API Version 1, which MongoDB guarantees will behave the same way, even as newer versions are released. This backward compatibility was crucial in maintaining uninterrupted service.
Here’s how we specified the API version in our Node.js application:
const { MongoClient } = require('mongodb');
async function main() {
const client = new MongoClient('mongodb://localhost:27017', {
useUnifiedTopology: true,
serverApi: { version: "1" } // Specify stable API version 1
});
try {
await client.connect();
const db = client.db('logisticsDB');
const shipments = db.collection('shipments');
// Find all active shipments using the stable API
const activeShipments = await shipments.find({ status: 'active' }).toArray();
console.log(activeShipments);
} finally {
await client.close();
}
}
main().catch(console.error);
Seamless Upgrades Without Fear
With the Stable API version locked in, we were able to upgrade MongoDB seamlessly, without the risk of breaking existing operations. By using API Version 1, we ensured that all queries, update operations, and MongoDB commands our logistics system relied on would continue to behave as expected.
Real-World Benefits of the Stable API
- Reduced Testing Time: Previously, every upgrade required extensive regression testing to ensure that none of our queries or write operations behaved unexpectedly. With the Stable API, we could focus our testing efforts solely on new features or parts of the application we intentionally modified. The bulk of the application, which relied on existing MongoDB behavior, was effectively safeguarded by the Stable API, drastically reducing testing time.
- Increased Development Speed: With the MongoDB Stable API, developers could concentrate more on building new features instead of worrying about how MongoDB version upgrades might affect their code. The API version acted as a contract, ensuring that their queries and updates wouldn’t suddenly break due to changes in the underlying database.
- Confidence in Production Upgrades: In real-time applications, downtime can lead to significant financial losses and reputational damage. Thanks to the Stable API, we could upgrade MongoDB with confidence, knowing that our mission-critical operations would continue to function as expected. This allowed us to execute production upgrades without extended maintenance windows or late-night panic.
Leveraging Stable Features and Future-Proofing
A significant advantage of using MongoDB’s Stable API is its ability to future-proof our operations. MongoDB continuously enhances its performance, security, and feature set, and the Stable API allowed us to upgrade our infrastructure and take advantage of these improvements without worrying about backward compatibility.
Example:
Handling count
Command Deprecation and ReintroductionIn earlier MongoDB versions (prior to 5.0), the count command was deprecated due to performance inefficiencies, particularly in sharded clusters. We transitioned to using countDocuments for better accuracy and performance in distributed environments.
However, starting with MongoDB 5.0.9 and MongoDB 6.0, the count
command was reintroduced in Stable API Version 1. This reintroduction provided us the flexibility to use count
in scenarios where performance concerns were minimal, such as smaller datasets, while continuing to rely on countDocuments
for more complex, sharded collections.
Why the Stable API is Critical for Database Operations
For any organization managing real-time or mission-critical systems, the Stable API in MongoDB is an indispensable tool. Here’s why:
- Backward Compatibility Across Versions: MongoDB’s Stable API guarantees backward compatibility across upgrades, allowing us to upgrade MongoDB with confidence that essential queries will behave as expected.
- Reduced Testing Time and Cost: By locking our clients’ operations to a specific API version, we significantly reduce the risk of breaking changes that could cause downtime. This is crucial for high-availability platforms that operate 24/7.
- Predictable System Behavior: The Stable API ensures that operations won’t suddenly start behaving differently after an upgrade, thereby reducing the risk of downtime and system disruption in production environments.
- Flexibility for Future Growth: As MongoDB continues to release new API versions, organizations can choose when to adopt new features, enabling them to grow and scale their systems without sacrificing stability.
Given these advantages, we have utilized MongoDB’s Stable API to maintain system stability and scalability for our clients. By locking our logistics platform to Stable API Version 1, we ensured that our queries and operations would continue to function reliably, even as we upgraded MongoDB to newer versions. This approach allowed us to scale with confidence, reduce testing costs, and prevent costly downtime, making MongoDB’s Stable API a critical tool for any large-scale, mission-critical application.
For further reading on enhancing your MongoDB skills, check out our other resources:
- Mastering Sorting in MongoDB: Arrays, Objects, and Collation
- Mastering Null Handling in MongoDB
- The Ultimate Guide to MongoDB TTL Indexes
If you are managing complex systems and looking for ways to future-proof your MongoDB infrastructure while minimizing risk, MongoDB’s Stable API should be an integral part of your strategy.
Ensure seamless upgrades and robust system stability with Mydbops' Managed, Consulting and Remote DBA Services. Contact us today to optimize your MongoDB environment for growth and success.