.png)
Sorting in MongoDB is a versatile and powerful feature, but it becomes more complex when working with arrays, embedded documents, and locale-sensitive string comparisons using collation. For MongoDB architects or advanced users, understanding how these sorting mechanisms function is critical to optimizing query performance and ensuring accurate data retrieval. This guide delves into the intricacies of array sorting, the significance of lexicographical order in embedded documents, and how collation supports language-specific and culturally sensitive sorting.
Sorting Arrays in MongoDB
Arrays in MongoDB offer flexibility, but their sorting behavior can sometimes yield unexpected results due to how MongoDB processes arrays during sorting. Unlike traditional databases, MongoDB doesn’t view an array as a single entity when performing sort operations. Instead, it treats each element within the array individually. This means that rather than sorting the entire array as one cohesive unit, MongoDB sorts based on the values of individual elements, which can lead to outcomes that might not be immediately intuitive.
How MongoDB handles array sorting
When sorting arrays:
- In descending order, MongoDB sorts by the largest element in the array.
- In ascending order, it sorts by the smallest element.
- When necessary, MongoDB uses the first element for further comparisons.
Examples and handling unexpected results
Let's take a closer look at how MongoDB handles sorting arrays when documents contain array fields. Consider the following set of documents in a collection, each with an array field x
:
{ x: [ 1, 11 ] }
{ x: [ 5 ] }
{ x: [ 4 ] }
{ x: [ 3 ] }
{ x: [ 2, 10 ] }
If we sort these documents based on the array x
in descending order using the following command:
db.collection.find().sort({ x: -1 });
MongoDB will return the documents in this order:
{ x: [ 1, 11 ] }
– Largest value is 11.{ x: [ 5 ] }
– Largest value is 5.{ x: [ 4 ] }
– Largest value is 4.{ x: [ 3 ] }
– Largest value is 3.{ x: [ 2, 10 ] }
– Largest value is 10, but it comes last.
At first glance, the last document { x: [ 2, 10 ] }
seems out of place, especially since 10 is larger than 3, 4, and 5. To understand why MongoDB sorted it this way, let's break down its sorting logic.
Why Does [2, 10] Come Last?
MongoDB prioritizes the largest element in each array when sorting in descending order. However, when arrays have similarly large elements, MongoDB looks at the first element in each array to break the tie. This means that even if an array contains a larger number later on, its position in the sorted order will depend on the value of its first element.
In this example, the array [2, 10]
is sorted last because MongoDB compares the first element, which is 2, against the first elements of the other arrays—5, 4, and 3. Since 2 is smaller than all of these, the array is placed at the end of the sorted list, even though it contains 10, a larger number than those in the other arrays.
Pro tip: Using aggregation to sort by specific array elements
To sort by a specific element in an array (e.g., always by the largest element), consider using the aggregation framework with $project and $max to control sorting more precisely.
db.collection.aggregate([
{ $project: { largest_value: { $max: "$x" }, x: 1 }},
{ $sort: { largest_value: -1 }}
]);
This ensures MongoDB sorts by the largest value in each array.
Sorting Embedded Documents
Sorting embedded documents in MongoDB isn't just about comparing values; MongoDB sorts these objects lexicographically, which means it first compares field names alphabetically before assessing the values within those fields. This approach can lead to seemingly unexpected results if the order of fields differs between documents.
Lexicographical Sorting
For example, take two documents that contain identical data but have different field orders:
{ a: 1, b: 1 }
{ b: 1, a: 1 }
Even though the values are the same, MongoDB will sort them differently. The document { a: 1, b: 1 }
will come before { b: 1, a: 1 }
because the field name "a" precedes "b" in alphabetical order.
Why Lexicographical Sorting Matters
If your documents have inconsistent field orders, MongoDB may return unpredictable or unexpected results when sorting. This is because MongoDB sorts embedded documents based on the lexicographical order of field names, rather than just the values they contain.
Maintaining a uniform field order helps MongoDB sort documents correctly and ensures that queries return results in the expected order, which is especially important in complex applications where sorting accuracy impacts functionality or user experience.
Pro tip: Ensuring consistent field order in documents
- To prevent inconsistencies, make sure that your MongoDB documents follow a consistent field order throughout your collections. This can be achieved by applying schema validation, which ensures that documents are structured uniformly.
- Additionally, using tools like Mongoose in Node.js can help enforce schema rules, automatically maintaining the correct field order when creating or updating documents.
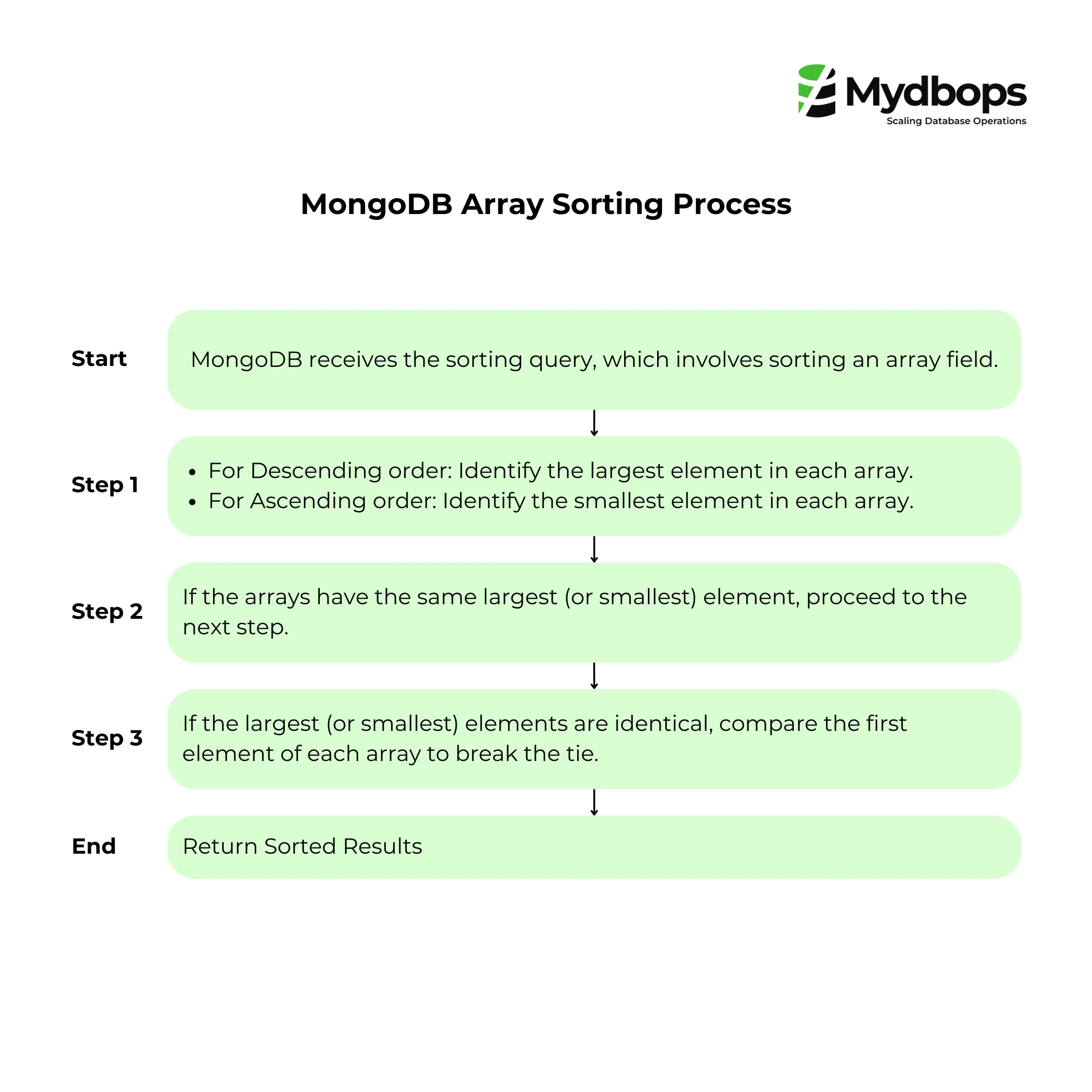
Collation: Locale-Specific Sorting
When working with applications that manage multilingual data, MongoDB’s collation feature is an invaluable asset. Collation allows you to apply locale-specific sorting rules that account for variations in case sensitivity, accent sensitivity, and character comparison. This ensures that your data is sorted in a way that is culturally and linguistically appropriate for your target audience.
Why Collation is Essential
By default, MongoDB sorts strings based on their Unicode code points, which may lead to results that do not conform to local language conventions. For example, in some languages, accented characters like "é" should be treated equivalently to "e," while in others, they are considered distinct characters. This discrepancy can result in sorting that feels inconsistent or incorrect to users familiar with those languages.
Example: Sorting Names for French Audiences
To demonstrate how to implement collation effectively, consider the case of sorting names for a French-speaking audience. You can create an index with locale-specific collation settings by using the following command:
db.collection.createIndex(
{ name: 1 },
{ collation: { locale: 'fr', strength: 1 } }
);
In this query:
- The
locale
is set to French (fr
), indicating that MongoDB will follow the sorting rules applicable to the French language. - The
strength
is configured to1
, allowing MongoDB to ignore both case and accent differences. As a result, characters like "é" and "e" are treated as equivalent, while "A" and "a" are considered equal as well. This ensures that your sorting aligns with the linguistic norms expected by your audience, enhancing the usability of your application.
Performance Implications of Sorting in MongoDB
Sorting operations in MongoDB, especially when dealing with large datasets, can significantly impact performance. Understanding the nuances of how sorting works is crucial for ensuring efficient query execution. Sorting requires resources, and if a dataset exceeds the memory limits, MongoDB will resort to writing temporary data to disk, leading to slower response times. To mitigate performance issues, it’s essential to implement best practices.
Performance Considerations for Sorting
- Sorting Arrays: Sorting large arrays can be performance-intensive. Whenever possible, try to reduce the size of arrays that need to be sorted or simplify their structure.
- Indexing: Creating indexes on fields that are frequently sorted will significantly improve query performance. MongoDB can use these indexes to avoid having to sort large datasets in memory.
- Collation Overhead: Using collation for locale-specific sorting adds overhead. While necessary for some applications, make sure to test and measure the performance impact of collation in your production environment.
Best Practices for Optimizing Sorting
- Use Aggregation for Complex Sorting: If your sorting involves complex structures (e.g., sorting by elements within arrays), use the aggregation pipeline to gain more control over how sorting is done.
- Schema Design: Ensure that embedded documents use a consistent field order to avoid unexpected results in lexicographical sorting.
- Limit Result Sets: Avoid sorting large result sets in queries without limits. Sorting a large dataset without limiting the result set can cause MongoDB to use additional memory and degrade performance.
- Test with Production Data: Always test the performance of sorting queries with production-sized data. This will help you identify bottlenecks and optimize indexing strategies before your application goes live.
In summary, understanding sorting in MongoDB is essential for developers seeking efficient data retrieval and optimal performance. Ensuring consistent field ordering, implementing appropriate indexing, and utilizing collation can help avoid common pitfalls and enhance application usability. Leveraging aggregation for complex sorting further improves control, while ongoing testing and optimization ensure your applications effectively meet user needs.
Struggling with MongoDB sorting or performance issues? Our team of MongoDB experts can help optimize your database with tailored solutions for array sorting, collation, and query performance. Get in touch with us today to boost your MongoDB performance with our managed and consulting services.